A Quick Sequence Diagram Review
Thomas J. Kennedy
Contents:
Consider a use case for a vending machine that accepts credit card payments
- A customer, Tom, swipes his credit card in the vending machine slot.
- The vending machine relays the card number over the network to a bank, requesting authorization for a small charge.
- The bank responds with an authorization number, indicating that the transaction is pre-approved.
- Tom selects a diet soda.
- The vending machine sends the charge (authorization number and the cost of the soda) to the bank.
- The vending machine dispenses the soda to Tom.
Let us start with the following diagram:
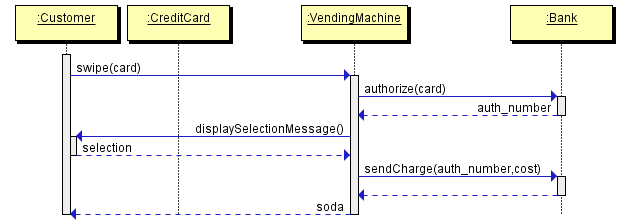
#
customer:Customer[a]
card:CreditCard[a]
machine:VendingMachine[a]
bank:Bank[a]
#
customer:soda=machine.swipe(card)
machine:auth_number=bank.authorize(card)
machine:selection=customer.displaySelectionMessage()
machine:bank.sendCharge(auth_number,cost)
First we replace the anonymous customer object with a named customer object:
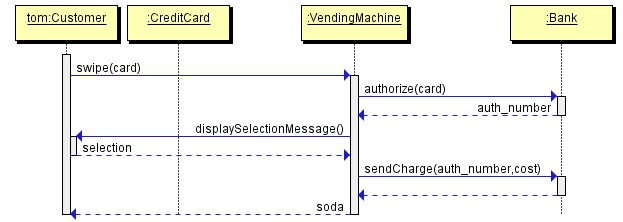
#
tom:Customer
card:CreditCard[a]
machine:VendingMachine[a]
bank:Bank[a]
#
tom:soda=machine.swipe(card)
machine:auth_number=bank.authorize(card)
machine:selection=tom.displaySelectionMessage()
machine:bank.sendCharge(auth_number,cost)
We then remove displaySelectionMessage()
and split the call to VendingMachine
:
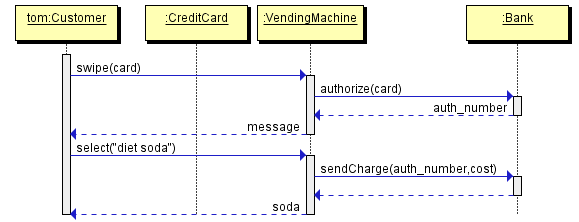
tom:Customer
card:CreditCard[a]
machine:VendingMachine[a]
bank:Bank[a]
#
tom:message=machine.swipe(card)
machine:auth_number=bank.authorize(card)
tom:soda=machine.select("diet soda")
machine:bank.sendCharge(auth_number,cost)
How would we modify this for the alternate case where Tom’s card is declined?
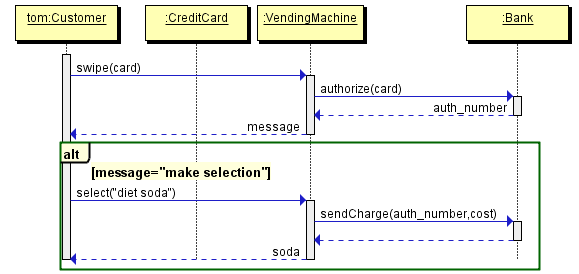
tom:Customer
card:CreditCard[a]
machine:VendingMachine[a]
bank:Bank[a]
#
tom:message=machine.swipe(card)
machine:auth_number=bank.authorize(card)
#
[c:alt message="make selection"]
tom:soda=machine.select("diet soda")
machine:bank.sendCharge(auth_number,cost)
[/c]